Http 请求开发
在 app 中对外进行 http 请求必须使用指定 API 来操作,否则会导致无法访问,app 的容器环境会把访问外部网络的防火墙打开,所以直接访问外部 url 是不通的。
spring boot 提供了 RestTemplate 来发送 http 请求。在 app 框架中,对 resttemplate 做了 http proxy 封装,所以在 app 中进行外部 url 请求需要使用 RestTemplate。
引入依赖
在需要用到 http 请求的模块中引入 spring-web 依赖:
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-web</artifactId>
</dependency>
使用示例
@Service
public class TestClient {
private final static Logger logger = LoggerFactory.getLogger(TestClient.class);
@Autowired
private RestTemplate restTemplate;
public void testHttpRequest() {
HttpHeaders requestHeaders = new HttpHeaders();
requestHeaders.add("key1", "values");
requestHeaders.add("key2", "ddd");
MultiValueMap<String, Object> params= new LinkedMultiValueMap<String, Object>();
params.add("key", "free");
params.add("appid", 0);
params.add("msg", "鹅鹅鹅");
HttpEntity<MultiValueMap<String, Object>> requestEntity = new HttpEntity<MultiValueMap<String, Object>>(params, requestHeaders);
Map<String, Object> map = new HashMap<>();
map.put("key", "free");
map.put("appid", 0);
map.put("msg", "鹅鹅鹅");
ResponseEntity<String> response = restTemplate.exchange("http://api.qingyunke.com/api.php?key=free&appid=0&msg=鹅鹅鹅", HttpMethod.POST, requestEntity, String.class);
MultiValueMap<String, Object> authorMap= new LinkedMultiValueMap<String, Object>();
authorMap.add("city", "北京");
String postForObject = restTemplate.getForObject("https://www.sojson.com/open/api/weather/json.shtml?city=北京", String.class);
MultiValueMap<String, Object> authorMap1= new LinkedMultiValueMap<String, Object>();
authorMap1.add("authorId", 2);
String postForObject1 = restTemplate.postForObject("https://www.baidu.com", null, String.class);
return ResultDTO.success();
}
}
需要申请域名配置
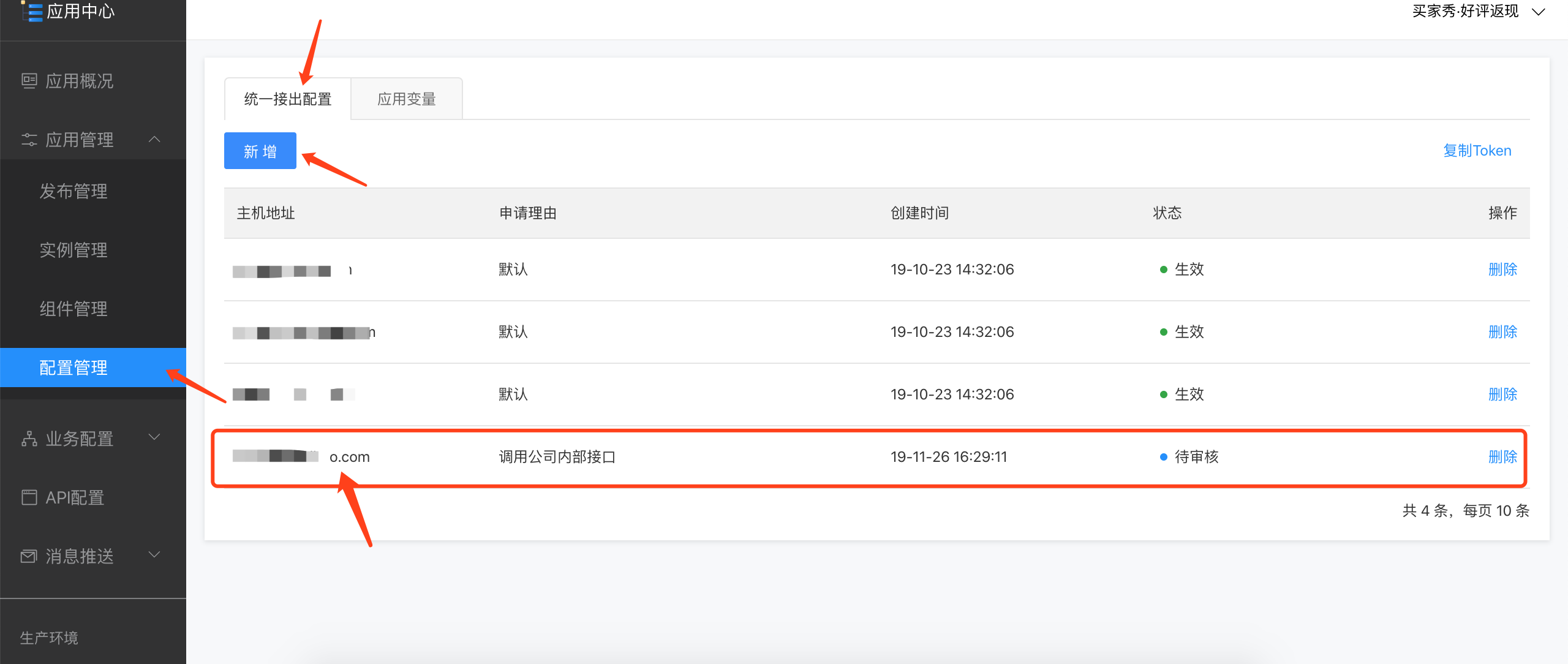